Java之路何其漫长(java流程控制)
Scanner对象
基本语法:
1
| Scanner s = new Scanner(System.in);
|
通过Scanner类的next()与nextLine()方法获取的字符串,在读取前我们一般需要使用hasNext()与hasextLine()判断是否还有输入数据
使用nextLine接收
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import java.util.Scanner;
public class Test{ public static void main(String[] args){ Scanner scanner = new Scanner(System.in); System.out.println("使用nextline方式接收:");
if (scanner.hasNextLine()) { String str = scanner.nextLine(); System.out.println("输出内容是: "+str);
} scanner.close(); } }
|
使用next接收:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import java.util.Scanner;
public class Test{ public static void main(String[] args){ Scanner scanner = new Scanner(System.in); System.out.println("使用next方式接收:");
if (scanner.hasNext()) { String str = scanner.next(); System.out.println("输出内容是: "+str);
} scanner.close(); } }
|
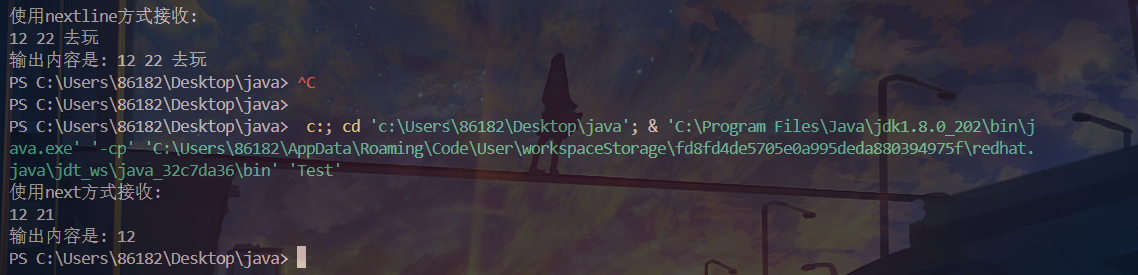
注意点:
1 2 3
| next():不能得到带有空格的字符串,以空白作为分隔符或者结束符
nextLine():以enter为结束符也就是说nextLine()方法返回的是输入回车之前的字符,也可以是空白
|
例题:
我们可以输入多个数字,并求其总和与平均数,没输入一个数字用回车确认通过输入非数字来结束输入并输出结果;
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| import java.util.Scanner;
public class Test{ public static void main(String[] args){ Scanner scanner = new Scanner(System.in); double sum = 0; int m = 0; while(scanner.hasNextDouble()){ double x = scanner.nextDouble(); m = m + 1; sum = sum + x; } System.out.println(m + "个数的和为" + sum); System.out.println(m + "个数的平均值为" + (sum/m)); scanner.close(); }
}
|
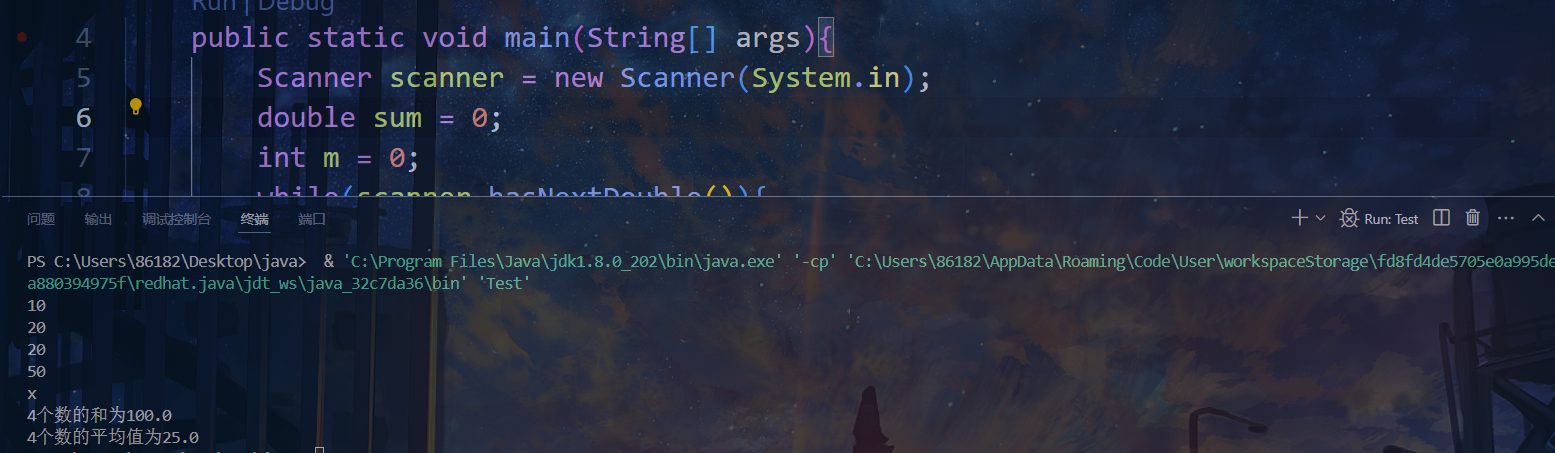
选择结构
- if单选择结构
- if双选择结构
- if多选择结构
- 嵌套的if结构
- switch多选择结构
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import java.util.Scanner;
public class Test{ public static void main(String[] args){ Scanner scanner = new Scanner(System.in); System.out.println("请输入成绩:"); int score = scanner.nextInt(); if (score<60) { System.out.println("及格"); }else{ System.out.println("不及格"); }
scanner.close(); }
}
|
1 2 3 4 5 6 7 8 9 10 11
| switch(expression){ case value1: break; case value2: break; case value3: break; default: }
|
循环结构
- 在java5中引入了一种主要用于数组的增强型for循环
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
while: int i = 0; int sum = 0; while(i<=100){ sum = sum + i; i++; }
do...while: int i = 0; int sum = 0; do{ sum = sum + i; i++ } while(i<=100);
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| for: for(初始化;布尔表达式;更新){ } 尝试打印九九乘法表
public class Test{ public static void main(String[] args){ for(int j = 1; j <= 9; j++){ for (int i = 1; i <= j; i++) { System.out.print(j+"*"+i+"="+(j*i) + '\t'); } System.out.println(); } }
}
|
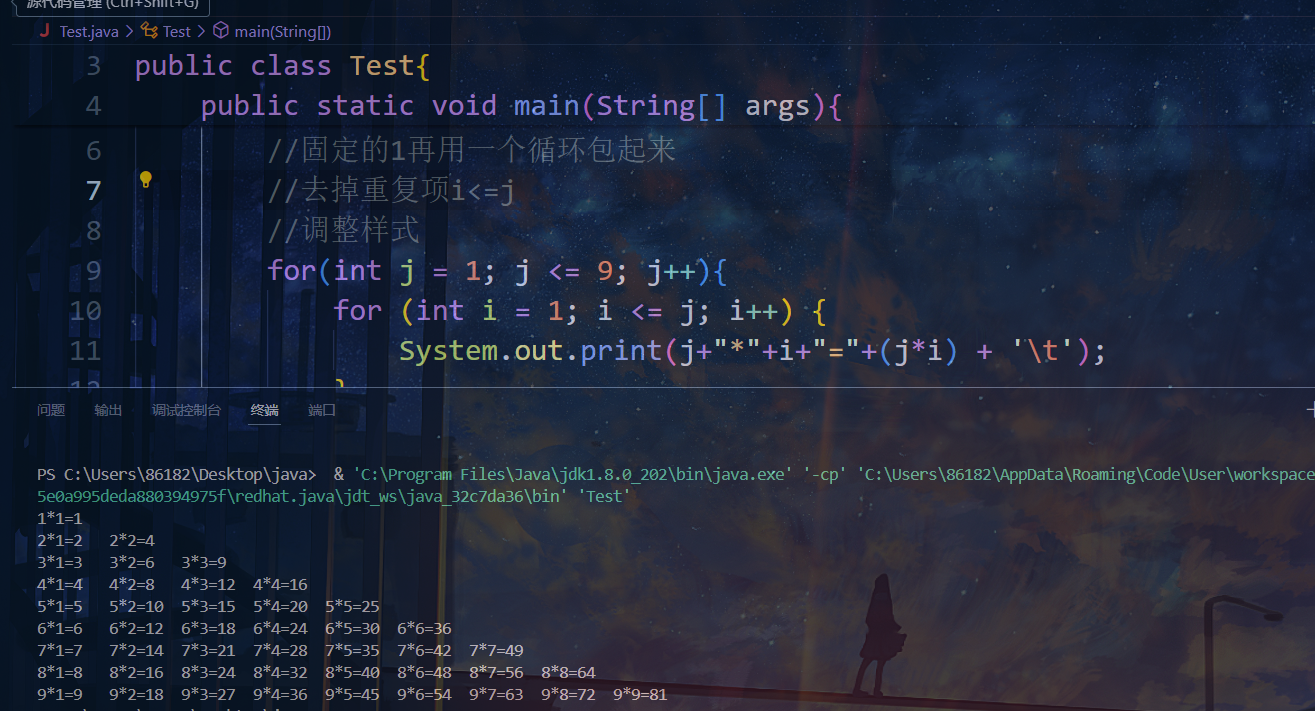